Contract Storage
The most common way for interacting with a contract’s storage is through storage variables. As stated previously, storage variables allow you to store data that will be stored in the contract's storage that is itself stored on the blockchain. These data are persistent and can be accessed and potentially modified anytime once the contract is deployed.
Storage variables in Starknet contracts are stored in a special struct called Storage
:
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
The Storage
struct is a struct like any other, except that it must be annotated with the #[storage]
attribute. This annotation tells the compiler to generate the required code to interact with the blockchain state, and allows you to read and write data from and to storage. Moreover, this allows you to define storage mappings using the dedicated Map
type.
Variables declared in the Storage
struct are not stored contiguously but in different locations in the contract's storage. The storage address of a particular variable is determined by the variable's name, and the eventual keys of the variable if it is a mapping.
Addresses of Storage Variables
The address of a storage variable is computed as follows:
-
If the variable is a single value, the address is the
sn_keccak
hash of the ASCII encoding of the variable's name.sn_keccak
is Starknet's version of the Keccak256 hash function, whose output is truncated to 250 bits. -
If the variable is composed of multiple values (i.e., a tuple, a struct or an enum), we also use the
sn_keccak
hash of the ASCII encoding of the variable's name to determine the base address in storage. Then, depending on the type, the storage layout will differ. See the "Storing Custom Types" section. -
If the variable is a mapping with a key
k
, the address of the value at keyk
ish(sn_keccak(variable_name),k)
, where ℎ is the Pedersen hash and the final value is taken modulo \( {2^{251}} - 256\). If the key is composed of more than onefelt252
, the address of the value will beh(...h(h(sn_keccak(variable_name),k_1),k_2),...,k_n)
, withk_1,...,k_n
being allfelt252
that constitute the key. In the case of mappings to complex values (e.g., tuples or structs), then this complex value lies in a continuous segment starting from the address calculated with the previous formula. Note that 256 field elements is the current limitation on the maximal size of a complex storage value.
You can access the base address of a storage variable by calling the address
attribute on the variable, which returns a felt252
value.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Accessing Storage Variables
Variables stored in the Storage
struct can be accessed and modified using the read
and write
functions, respectively. As previously mentioned, you can access their address in storage through the address
field. All these functions are automatically generated by the compiler for each storage variable.
Accessing full types
To read the value of the owner
storage variable, which is of type Person
, we call the read
function on the owner
variable, passing in no arguments.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
To write a new value to the storage slot of a storage variable, we call the write
function, passing in the value as argument. Here, we only pass in the value to write to the owner
variable as it is a simple variable.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Accessing mappings
To read the value of the storage variable names
, which is a mapping from ContractAddress
to felt252
, we first need to retrieve the entry path for the specific key in the mapping. We do this by calling the entry
method on the names
variable, passing in the address
as a parameter. This gives us access to the specific entry in the mapping.
Once we have the entry path, we can call the read
function on it to retrieve the stored value.
If the mapping had more than one key, we would chain multiple entry
calls before the final read
, passing in each key as a parameter.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Similarly, to write a value in a storage mapping, we need to retrieve the storage entry path corresponding to the key, just as we did when reading the value. Once we have this entry path, we can call the write
function on it with the value to write. If the mapping had more than one key, we would chain multiple entry
calls with respective keys before the final write
, passing in value as a parameter.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Accessing Individual Storage Members
When working with structs, instead of calling read
and write
on the struct variable itself, you can call read
and write
on the individual members of the struct. This allows you to access and modify the values of the struct members directly, minimizing the amount of storage operations performed. In the following example, the owner
variable is of type Person
. Thus, it has one attribute called name
, on which we can call the read
and write
functions to access and modify the value of the name
attribute.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Storing Custom Types
The Store
trait, defined in the starknet::storage_access
module, is used to specify how a type should be stored in storage. In order for a type to be stored in storage, it must implement the Store
trait. Most types from the core library, such as unsigned integers (u8
, u128
, u256
...), felt252
, bool
, ByteArray
, ContractAddress
, etc. implement the Store
trait and can thus be stored without further action.
But what if you wanted to store a type that you defined yourself, such as an enum or a struct? In that case, you have to explicitly tell the compiler how to store this type.
In our example, we want to store a Person
struct in storage, which is only possible by implementing the Store
trait for the Person
type. This can be simply achieved by adding a #[derive(starknet::Store)]
attribute on top of our struct definition. Note that all the members of the struct need to implement the Store
trait.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
Similarly, Enums can only be written to storage if they implement the Store
trait, which can be trivially derived as long as all associated types implement the Store
trait.
use starknet::ContractAddress;
#[starknet::interface]
pub trait INameRegistry<TContractState> {
fn store_name(
ref self: TContractState, name: felt252, registration_type: NameRegistry::RegistrationType
);
fn get_name(self: @TContractState, address: ContractAddress) -> felt252;
fn get_owner(self: @TContractState) -> NameRegistry::Person;
fn get_owner_name(self: @TContractState) -> felt252;
}
#[starknet::contract]
mod NameRegistry {
use starknet::{ContractAddress, get_caller_address, storage_access};
use starknet::storage::{Map, StoragePathEntry};
#[storage]
struct Storage {
names: Map::<ContractAddress, felt252>,
owner: Person,
registration_type: Map<ContractAddress, RegistrationType>,
total_names: u128,
}
#[event]
#[derive(Drop, starknet::Event)]
enum Event {
StoredName: StoredName,
}
#[derive(Drop, starknet::Event)]
struct StoredName {
#[key]
user: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub struct Person {
address: ContractAddress,
name: felt252,
}
#[derive(Drop, Serde, starknet::Store)]
pub enum RegistrationType {
finite: u64,
infinite
}
#[constructor]
fn constructor(ref self: ContractState, owner: Person) {
self.names.entry(owner.address).write(owner.name);
self.total_names.write(1);
self.owner.write(owner);
}
// Public functions inside an impl block
#[abi(embed_v0)]
impl NameRegistry of super::INameRegistry<ContractState> {
fn store_name(ref self: ContractState, name: felt252, registration_type: RegistrationType) {
let caller = get_caller_address();
self._store_name(caller, name, registration_type);
}
fn get_name(self: @ContractState, address: ContractAddress) -> felt252 {
self.names.entry(address).read()
}
fn get_owner(self: @ContractState) -> Person {
self.owner.read()
}
fn get_owner_name(self: @ContractState) -> felt252 {
self.owner.name.read()
}
}
// Standalone public function
#[external(v0)]
fn get_contract_name(self: @ContractState) -> felt252 {
'Name Registry'
}
// Could be a group of functions about a same topic
#[generate_trait]
impl InternalFunctions of InternalFunctionsTrait {
fn _store_name(
ref self: ContractState,
user: ContractAddress,
name: felt252,
registration_type: RegistrationType
) {
let total_names = self.total_names.read();
self.names.entry(user).write(name);
self.registration_type.entry(user).write(registration_type);
self.total_names.write(total_names + 1);
self.emit(StoredName { user: user, name: name });
}
}
// Free function
fn get_owner_storage_address(self: @ContractState) -> felt252 {
self.owner.address
}
}
You might have noticed that we also derived Drop
and Serde
on our custom types. Both of them are required for properly serializing arguments passed to entrypoints and deserializing their outputs.
Structs Storage Layout
On Starknet, structs are stored in storage as a sequence of primitive types.
The elements of the struct are stored in the same order as they are defined in the struct definition. The first element of the struct is stored at the base address of the struct, which is computed as specified in "Addresses of Storage Variables" section and can be obtained by calling var.address()
, and subsequent elements are stored at addresses contiguous to the first element.
For example, the storage layout for the owner
variable of type Person
will result in the following layout:
Fields | Address |
---|---|
name | owner.address() |
address | owner.address() +1 |
Note that tuples are similarly stored in contract's storage, with the first element of the tuple being stored at the base address, and subsequent elements stored contiguously.
Enums Storage Layout
When you store an enum variant, what you're essentially storing is the variant's index and eventual associated values. This index starts at 0 for the first variant of your enum and increments by 1 for each subsequent variant.
If your variant has an associated value, this value is stored starting from the address immediately following the address of the index of the variant.
For example, suppose we have the RegistrationType
enum with the finite
variant that carries an associated limit date, and the infinite
variant without associated data. The storage layout for the finite
variant would look like this:
Element | Address |
---|---|
Variant index (0 for finite) | registration_type.address() |
Associated limit date | registration_type.address() + 1 |
while the storage layout for the infinite
would be as follows:
Element | Address |
---|---|
Variant index (1 for infinite) | registration_type.address() |
Storage Mappings
Storage mappings are similar to hash tables in that they allow mapping keys to values. However, unlike a typical hash table, the key data itself is not stored - only its hash is used to look up the associated value in the contract's storage. Mappings do not have a concept of length or whether a key/value pair is set. All values are by default set to 0. The only way to remove an entry from a mapping is to set its value to the default zero value.
Mappings are only used to compute the location of data in the storage of a contract given certain keys. They are thus only allowed as storage variables. They cannot be used as parameters or return parameters of contract functions, and cannot be used as types inside structs.
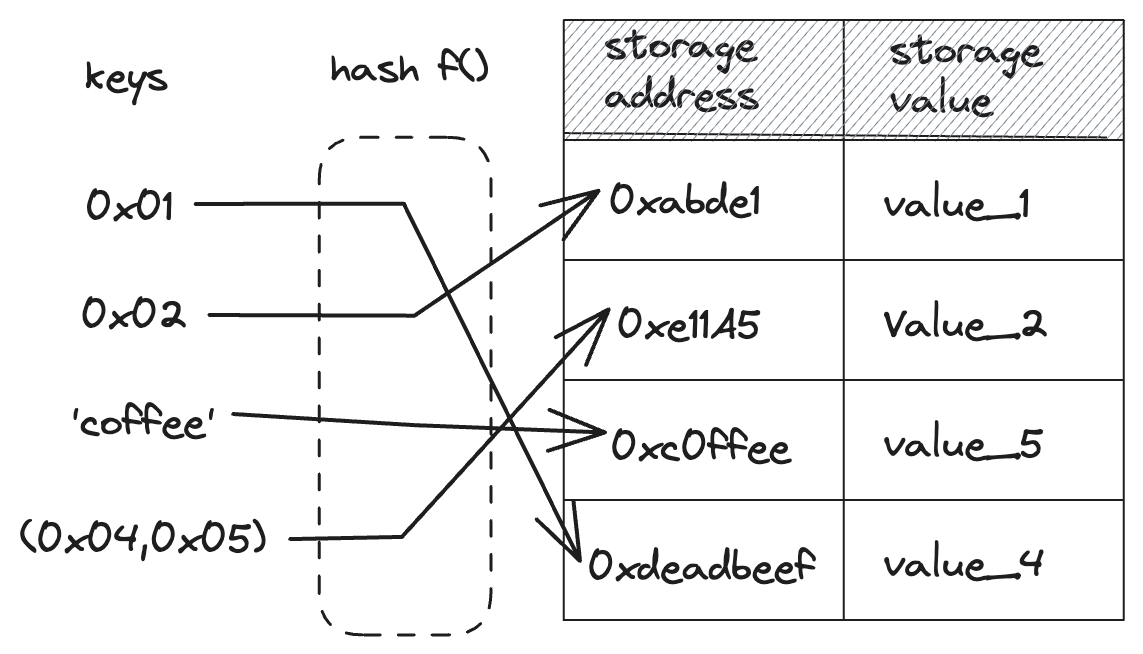
To declare a mapping, use the LegacyMap
type enclosed in angle brackets <>
,
specifying the key and value types.
Note: You might encounter LegacyMap
in older code or documentation. This was the previous mapping type used in Cairo contracts, but it has been deprecated in favor of the more flexible Map
type. If you come across LegacyMap
, it's recommended to update it to Map
that provides more control over storage, as they have identical storage layouts allowing for a safe migration.
You can also create more complex mappings with multiple keys. You can find in Listing 14-2 the popular allowances
storage variable of the ERC20 Standard which maps an owner
and an allowed spender
to their allowance
amount using multiple keys passed inside a tuple:
#[storage]
struct Storage {
allowances: Map<ContractAddress, Map<ContractAddress, u256>>,
}
Listing 14-2: Storing a mapping with multiple keys inside a tuple
The address in storage of a variable stored in a mapping is computed according to the description in the "Addresses of Storage Variables" section.
If the key of a mapping is a struct, each element of the struct constitutes a key. Moreover, the struct should implement the Hash
trait, which can be derived with the #[derive(Hash)]
attribute. For example, if you have a struct with two fields, the address will be h(h(sn_keccak(variable_name),k_1),k_2)
modulo \( {2^{251}} - 256\), where k_1
and k_2
are the values of the two fields of the struct.
Similarly, in the case of a nested mapping using a tuple as key, such as Map<ContractAddress, Map<ContractAddress, u8>>,
, the address will be computed in the same way, with each element of the tuple being a key: h(h(sn_keccak(variable_name),k_1),k_2)
.